Using the PayPal REST #API in C#
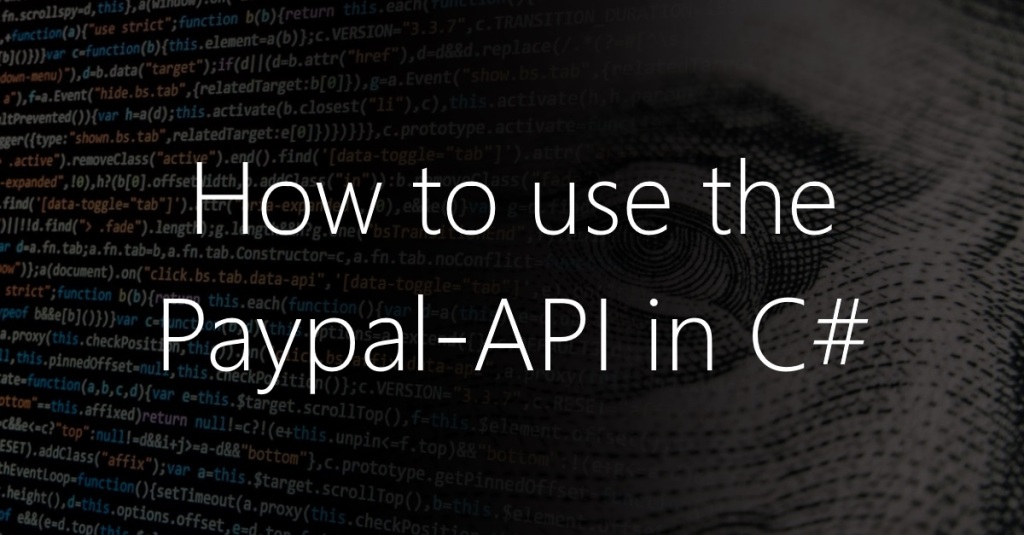
Some of this code requires custom-built libraries, so it’s not a great reference, but conceptually, you should be able to see how to use the PayPal REST API in C# using this technique
First, Authentication, you need to get a bearer token before you can all any interesting API endpoints;
private static string Authenticate(Environment environment)
{
var credential = Sandbox;
if (environment == Environment.LIVE) credential = Live;
var auth = Convert.ToBase64String(Encoding.UTF8.GetBytes(
credential.UserName + ":" + credential.Password));
var http = new HTTPRequest
{
HeaderHandler = h => new NameValueCollection
{
{ "Authorization", "Basic " + auth }
}
};
var apiAuthEndpoint = "https://api-m.sandbox.paypal.com/v1/oauth2/token";
if (environment == Environment.LIVE)
{
apiAuthEndpoint = "https://api-m.paypal.com/v1/oauth2/token";
}
const string strGrant = "grant_type=client_credentials";
var authResponse = http.Request(apiAuthEndpoint, "POST", strGrant);
var jAuthResponse = JObject.Parse(authResponse);
var token = jAuthResponse["access_token"] + "";
return token;
}
Once you have a token, then you can create an order, which in effect gives you a URL to send the user to, and an ID that you should keep for future reference;
var token = Authenticate(environment);
var intPackageAmount = 100;
var packageCurrency = "USD";
var intent = new
{
intent = "CAPTURE",
purchase_units = new[]
{
new
{
amount = new
{
currency_code = packageCurrency,
value = intPackageAmount
}
}
},
payment_source = new
{
paypal = new
{
experience_context = new
{
payment_method_preference = "UNRESTRICTED",
landing_page = "LOGIN",
user_action = "PAY_NOW",
return_url = domain + "/payments/paypalOrderComplete.aspx",
cancel_url = domain
}
}
}
};
var json = JsonConvert.SerializeObject(intent, Formatting.Indented);
var apiEndpoint = "https://api-m.sandbox.paypal.com/v2/checkout/orders";
if (environment == Environment.LIVE)
{
apiEndpoint = "https://api-m.paypal.com/v2/checkout/orders";
}
var http = new HTTPRequest
{
HeaderHandler = h => new NameValueCollection
{
{ "Authorization", "Bearer " + token }
},
ContentType = "application/json"
};
var orderSetupJson = http.Request(apiEndpoint, "POST", json);
var jOrderSetup = JObject.Parse(orderSetupJson);
var paypalId = jOrderSetup["id"] + "";
var linksArray = jOrderSetup["links"] as JArray;
if (linksArray == null) throw new Exception("Paypal Order setup failed");
var url = "";
foreach (var link in linksArray)
{
if (link["rel"] + "" != "payer-action") continue;
url = link["href"] + "";
break;
}
The Option that said “unrestricted”, helped later on with the capture part.
Once you’ve sent the user to the URL provided by paypal, and it’s returned to your return url, it should also provide you with a token in the Querystring, which matches the id of the order. – I’ve omitted the bit where this is held in a database while the user makes the payment.
Once it comes back, you need to capture the payment as follows;
// Capture payment
var apiCaptureEndpoint = "https://api-m.paypal.com/v2/checkout/orders/{0}/capture";
if (environment == Environment.LIVE)
{
apiCaptureEndpoint = "https://api-m.paypal.com/v2/checkout/orders/{0}/capture";
}
apiCaptureEndpoint = string.Format(apiCaptureEndpoint, paypalOrderId);
var captureResponse = http.Request(apiCaptureEndpoint, "POST", "");
var jCaptureResponse = JObject.Parse(captureResponse);
status = jCaptureResponse["status"] + "";
Status should be “COMPLETED” after this process is completed, and the money should be transferred.
Test first on sandbox before going live.